Like most programming languages, PowerShell differentiates between integers and strings. While it’s easy to distinguish between strings and numbers, complex scripts may require combining the two. This raises the question of how to handle calculations when you need to work with a number that’s stored as a string.
What Happens If You Add Strings?
In situations like this, you have several options. Let’s consider a scenario where you have two string variables, $A and $B, and you need to add their values. If you simply type $A + $B, you will end up with a concatenation of those values. For example, if $A is 1 and $B is 3, $A + $B would result in 13, not 4. This happens because $A and $B are treated as strings, so adding them together just combines them as $A (1) + $B (3) is the same as $A $B, which results in 13.
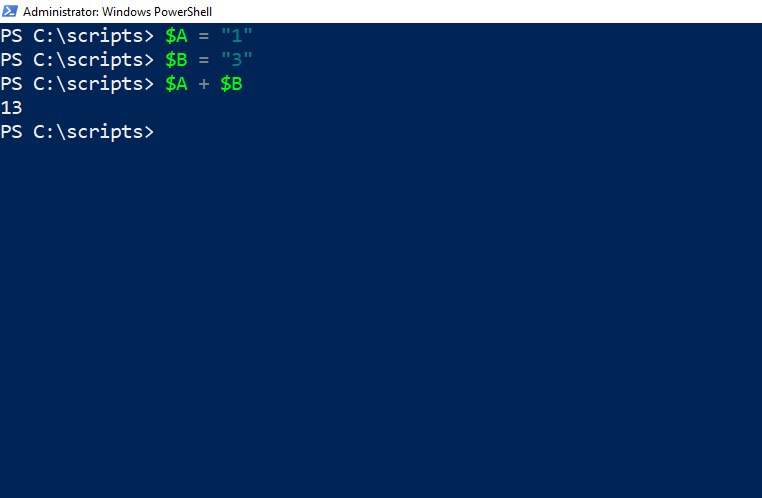
Figure 1. You can’t add strings together, even when the string variables contain numbers.
Convert the Strings to Numbers Using [Int]
But what if we need to add these two numbers together rather than just performing a string join (i.e., concatenating them)? The simplest way to deal with this is by typing [Int] before each variable name. This forces PowerShell to treat the values as integers rather than strings.
For example, you might type:
[Int]$A + [Int]$B
As you can see in Figure 2, following this approach will produce the expected result.
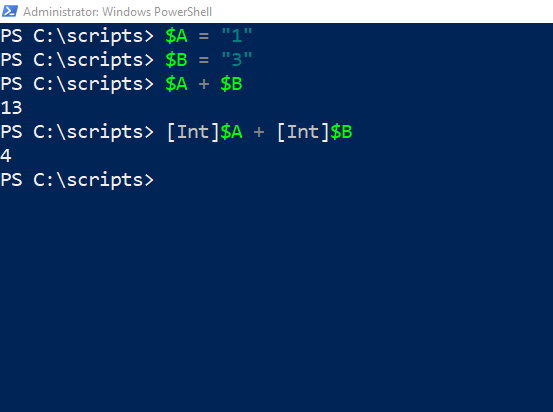
Figure 2. You can force PowerShell to treat string data as numerical data.
Evaluate the Expression
Converting string data into numerical format is, hands down, the simplest solution. Even so, the method that I just demonstrated doesn’t always work. For example, I recently built a basic calculator application in PowerShell. The application operates by creating a string consisting of user input. Each time a user clicks on a calculator key, the key’s value is added to the string.
At first, it might seem odd to treat calculator input as a string when calculators deal solely with numbers. The reason behind this approach is that operators (such as +, -, *, and /) are not numerical values. Therefore, adding a plus sign to a numerical variable would not be an option. Consequently, when the user clicks on the “equals” key, the string containing the user’s input typically includes a mixture of numbers and operators. For instance, the string might look like this: “123+456”
You can’t just convert “123+456" into numerical format because it contains an operator. As such, there are a couple of options for handling a string like this.
One option is to parse the string and use character manipulation to extract the individual elements (e.g., number 123, the plus sign, and the number 456). While this approach is valid, it involves some tricky programming.
A more elegant solution is to treat the string as an expression to be evaluated. You can do this by using PowerShell’s Invoke-Expression command in conjunction with the string’s contents.
Such an operation might look like this:
$A=”123+456” Invoke-Expression $A
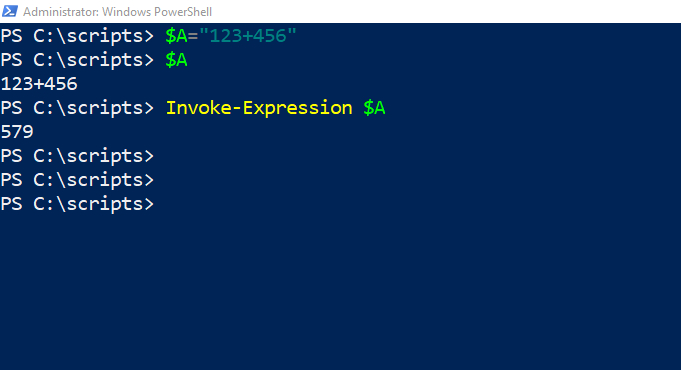
Figure 3. You can use the Invoke-Expression cmdlet to evaluate a string.
What If Invoke-Expression Fails?
The Invoke-Expression method I demonstrated is really straightforward. However, when dealing with a complex script, you may encounter situations where the Invoke-Expression command fails to produce results. In such cases, it often indicates that something might be wrong with your string. For example, you might not be dealing with true string data, or the string may contain invisible extra characters. To address this, I would like to introduce a technique.
The first step is to verify the variable’s type. The easiest way to do this is by appending .GetType() to the variable name. Check the resulting Name column to determine the variable’s type.
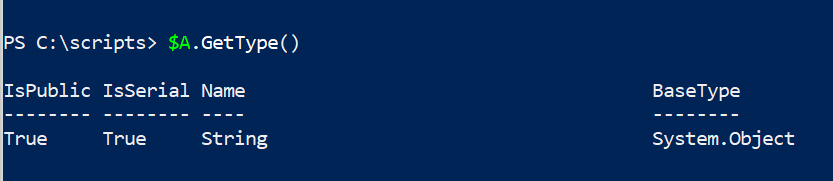
Figure 4. The Name column indicates that this is a string variable.
You can also check the variable’s length to ensure there are no hidden spaces at the beginning or end. Take, for instance, my $A variable, which contains the string “123+456”. This variable appears to have seven visible characters, with six of them being numbers and one being the operator (the plus sign). To check for the presence of extra, unseen characters, simply type the variable name and append .Length. You can see what this looks like in Figure 5.
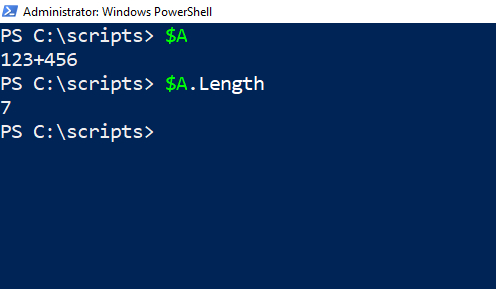
Figure 5. My variable contains seven characters.
If you encounter an unexpected result, it’s a signal to investigate why extra characters have found their way into your string. Once you eliminate these extra characters, you should be able to evaluate the expression correctly.
In case you can’t pinpoint the source of the extra characters, you can use the Trim operation to remove any leading or trailing spaces. For example, if $A contained extra characters, I could remove them by typing:
$B=$A.Trim() $A=$B
You can see what this looks like in Figure 6.
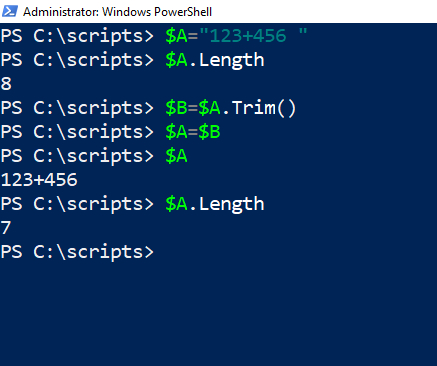
Figure 6. I have used Trim to remove the extra spaces from my string.
Another reason why Invoke-Expression may fail is that, when used inside a script, it might not always produce visible output. A better approach is to map the Invoke-Expression to a variable and then tell PowerShell to display the variable.
Here is an example of how to do this:
$A=”123+456” $Answer=Invoke-Expression $A $Answer
About the author
